This multi-part React tutorial will be covering how to use Mapbox to:
- display a map
- search an address
- add markers
- display a popup when clicking on a marker
and even removing a marker.
To not overwhelm people with a bunch of code, I will be splitting it up into three parts:
- Part 1: display a map and using a geocoder to look up addresses
- Part 2: Displaying a marker on a Mapbox map
- Part 3: Adding popups and removing markers on a Mapbox map
We will be using create-react-app for our base React app and using the react-map-gl and react-mapbox-gl-geocoder libraries for our map and geocoder respectively. As an option, I will be using reactstrap for styling (in case you are unfamiliar, reactstrap is Bootstrap for React).
Step 1. Create project and install dependencies
First, we will start by setting up our project.npx create-react-app mapbox-project
cd mapbox-project
npm i react-map-gl react-mapbox-gl-geocoder bootstrap reactstrap
npm start
Step 2. Get a Mapbox key
In order to use Mapbox, you will need an API key. Go to https://www.mapbox.com/ and click on Sign In.
Under the signin form, click on Sign Up for Mapbox. Once you are done signing up, you should be taken to your dashboard. If you weren’t automatically given a token, you can create one by clicking Create a token.
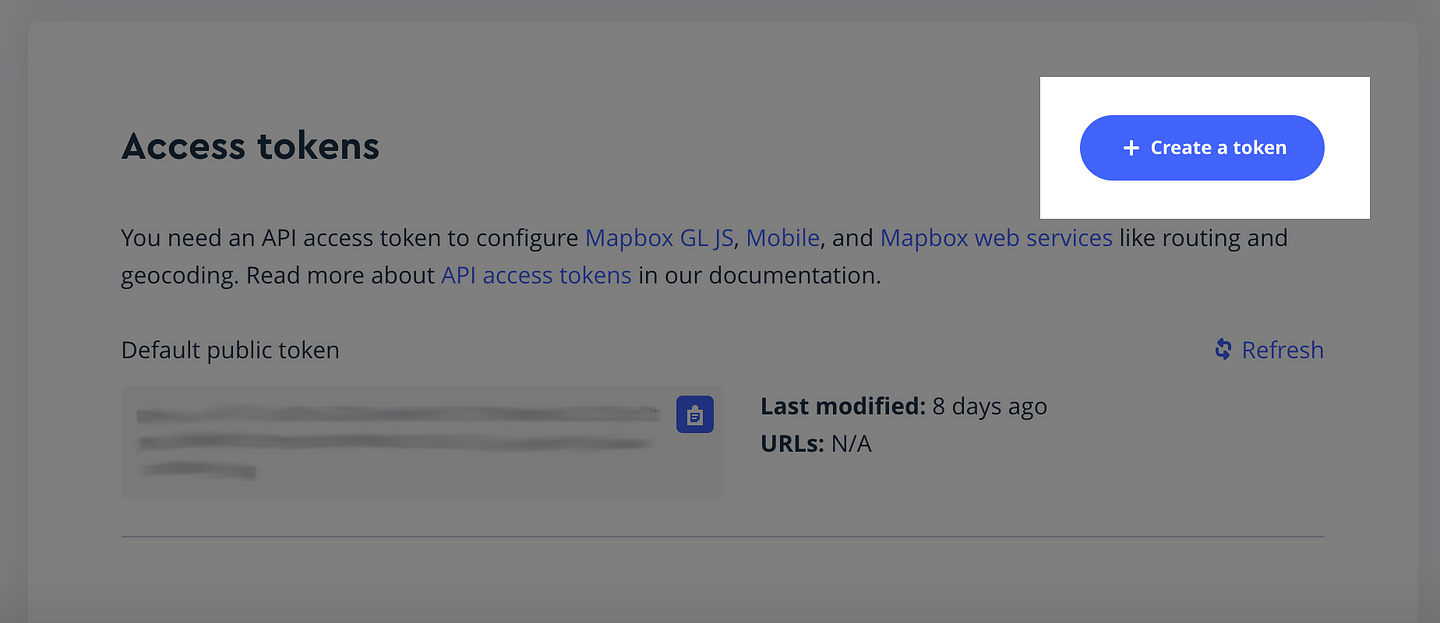
Step 3. Create a React Map component
Now that we have our API key, let’s start coding. First, we will focus on simply displaying a map thanks to the react-map-gl library. It comes with a ReactMapGL
component which accepts a series of props.
The obvious one is your API key but it also include:
- the
viewport
(the latitude/longitude coordinates to center the map + zoom) mapStyle
(Mapbox offers a series of different styles for their map including streets but also light, dark, satellite, …)onViewportChange
(when the user drags the map, the viewport is updated)
I am also going to include width and height to better display it.
After saving, you should see a map centered to your viewport. Fairly straightforward!
Step 4. Create a React Geocoder component
Let’s continue by adding our Geocoder. Once again, we get a nice out-of-the-box component thanks to react-mapbox-gl-geocoder.
import Geocoder from 'react-mapbox-gl-geocoder';
Like ReactMapGL
, it takes your Mapbox API key and viewport. It also accepts queryParams
which allows me to narrow the results returned to a particular country (for this tutorial, let’s say I am only interested in Canadian addresses).
<Geocoder
mapboxApiAccessToken={mapboxApiKey}
onSelected={this.onSelected}
viewport={viewport}
hideOnSelect={true}
value=""
queryParams={params}
/>
In addition, it accepts an onSelected
function which in our case, we want it to center our map to an address we selected.
onSelected = (viewport, item) => {
this.setState({
viewport
})
}
When you select an address, it returns the viewport and the item selected. By updating our state to this new viewport, the map will automatically re-center to this new location.
This is the end result:
Resources
The following libraries are mentioned in this tutorial:
react-map-gl: https://www.npmjs.com/package/react-map-gl
react-mapbox-gl-geocoder: https://www.npmjs.com/package/react-mapbox-gl-geocoder
bootstrap: https://www.npmjs.com/package/bootstrap
reactstrap: https://www.npmjs.com/package/reactstrap
And here is the link to Mapbox and it's examples: https://docs.mapbox.com/help/tutorials/
Conclusion
After saving, you will now have a map and a geocoder. This latter allows you to look up addresses and on selection, to update the map to center on this point.
This is a very nice start.
For more Mapbox tutorials in React: